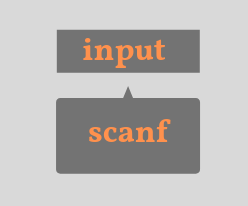
In this tutorial we will learn about accepting user input in C Programming. Computers perform three basic task, accept input, process data and print result as output. C language has many in-built functions to accept user input and print some result on output window. In this tutorial we will learn functions, which can be used to accept different types of user data.
scanf() Function in C Language
scanf() function in C language is used to accept formatted input from user and store it in a variable. scanf() function is in-built or predefined function of <stdio.h> header file. So, whenever you use this function, you need to include header file.
Example 1: Integer Input and Output in C.
#include<stdio.h>
int main()
{
int n,m,s;
printf("\nEnter First Number ");
scanf("%d",&n);
printf("\nEnter Second Number ");
scanf("%d",&m);
s=n+m;
printf("\nSum of %d and %d is %d\n",n,m,s);
return 0;
}
Output :
Enter First Number 11
Enter Second Number 22
Sum of 11 and 22 is 33
In the above C program, int is called data type and n,m,s are variables. We will discuss in detail about data type and variables in next chapter. Above Example accept two integer (whole number) value and print sum of given numbers.
- %d : It is a format for integer variable. It guides the function about type of information to be accept and print.
- & : It is address operator, it gets the address of variable and the value is stored in that address.
- scanf(“%d”,&n) : In this line of code, scanf() accept integer value and store it in variable n.
- printf(“\nSum of %d and %d is %d\n”,n,m,s) : Formatted print statement, print multiple values. Value of a variable will print in the position of %d, sequence wise.
We can accept more values as input in a single scanf() function.
printf("\nEnter First Number ");
scanf("%d",&n);
printf("\nEnter Second Number ");
scanf("%d",&m);
Note : Above line of code can also write as below, in single line.
printf("\nEnter Two Number ");
scanf("%d%d",&n,&m);
There are many other functions to accept input in C Programming, getch(), getchar(), gets() and scanf().