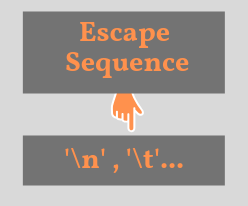
Escape Sequence or Escape characters are special characters start with
Popular Escape Sequence in C Language.
\n To change the line.
\t For Tabbed space between two characters or string.
Example 1: Both printf() statement will print in same line.
#include<stdio.h>
int main()
{
printf("Coder");
printf("Mantra");
return 0;
}
Output : CoderMantra
Example 2: Both printf() statement will print in different line because of \n.
#include<stdio.h>
int main()
{
printf("Coder");
printf("\nMantra");
return 0;
}
Output : Coder
Mantra
Example 3: Tabbed space (Tab key on
#include<stdio.h>
int main()
{
printf("Coder");
printf("\tMantra");
return 0;
}
Output : Coder Mantra
Example 4: Another Example of \n and \t.
#include<stdio.h>
int main()
{
printf("________________\n");
printf("|\t\t|\n");
printf("|\tHello \t|\n");
printf("|\t\t|\n");
printf("----------------\n");
return 0;
}
Output :
________________
| |
| Hello |
| |
----------------
List of Important Escape Sequences in C Programming.
\n To change the line
\t For Tabbed space between two character or string.
\b Backspace.
\r Carriage return (moves the cursor in the begining of line).
\ To print backslash ().
\" To print Quotation mark.
\' To print single inverted comma.