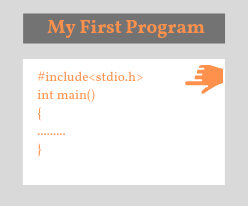
First C program to explain code and basic structure of C language.
#include<stdio.h>
int main()
{
printf("Hello World Program");
return 0;
}
Output : Hello World Program
Whenever we run this C language example, it will print Hello World Program as output. We will learn every line of the code step by step.
#include : This is called pre processor directive. It gives instruction to the compiler to include header file.
<stdio.h> : Standard Input Output header or library file includes some standard functions like
#include<stdio.h> : Instruction for compiler to include header file stdio.h to use
main() : main() is the first function called by the operating system as
int main() : int before main() is called return type of function, we will discuss it later in function chapter.
{ } : The opening and closing braces { }, is starting and ending point of function and also called body of main() function. All statement must be within braces.
printf() : The printf() function is used to print anything on the output window. This library function is define in header file, That’s why we need to include this.
return 0 : It is success message for operating system. If the code return 0 means program worked fine and if it return non zero to operatting system, means exited due to error in C code.
Semicolon ( ; ) : Can you notice semicolon(;) at the end of