Java array is a collection of multiple data items but of the same data type. In this tutorial, we will learn how to create, store and show data of an array in Java.
What is an Array?
- The array is a data structure.
- It can store multiple data but of the same type.
- Elements of the array stored at the consecutive memory location.
- Each element of an array is identified by an index (Key).
- Index of the array always starts with the zero (0).
Types of Array in Java.
- Single Dimensional
- Double Dimensional
Single Dimensional Array.
The single-dimensional array in Java store data in a linear format. We can assume array as a row with a single name but different index number (start with zero).
How to Declare Single Dimensional Array.
In this below array syntax, we are using int type, but we can declare an array of any data type in the same way. We can declare the array in java in three ways.
int n[]=new int[6];
int[] n=new int[6];
int n[];
n=new int[6];
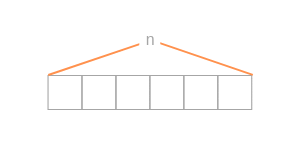
In the above picture, the size of the array is 6. Address of the first element is n[0] and address of the last element is n[5].
Example: How to accept values in an array and print them?
import java.util.*;
class Example
{
public static void main(String arg[])
{
//declare an array
int n[]=new int[5];
int i;
//Scanner object for input
Scanner in=new Scanner(System.in);
//accept user input in array
for(i=0; i<5; i++)
{
System.out.println("Enter a number");
n[i]=in.nextInt();
}
//display value of an array
for(i=0; i<5; i++)
{
System.out.println(n[i]);
}
}
}
//Output: In the above example program will accept 5 numbers in array and second loop will print all the values.
Double Dimensional Array.
The double dimensional array is represented as a table in Java, that is a combination of rows and columns. It is also known as matrix and index of the rows and columns always start with the zero(0).
How to Declare Double Dimensional Array?
Like a single-dimensional array, double dimensional array in Java is also declared in three ways.
int n[][]=new int[3][3];
int[][] n=new int[3][3];
int n[][];
n=new int[3][3];
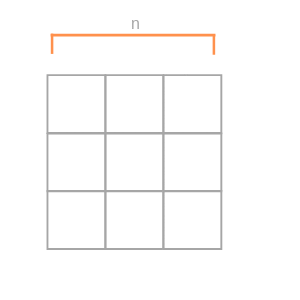
In the above picture, the matrix is of 3 rows and 3 columns. Address of the first cell is n[0][0] and address of the first row and the last column is n[0][2].
Example: Program in Java for matrix representation.
import java.util.*;
class Example
{
public static void main(String arg[])
{
//declare an matrix
int n[][]=new int[3][3];
int i, k;
//Scanner object for input
Scanner in=new Scanner(System.in);
//accept user input in array
for(i=0; i<3; i++)
{
for(k=0; k<3; k++)
{
System.out.println("Enter a number");
n[i][k]=in.nextInt();
}
}
//display in matrix format
for(i=0; i<3; i++)
{
for(k=0; k<3; k++)
{
System.out.print(n[i][k]);
}
System.out.println();
}
}
}
Loop Through an Array using For-Each.
For-Each loop is an enhanced for loop, that is used with collection and arrays.
class Example
{
public static void main(String arg[])
{
//declare and assign values in array
String colors[]={"Red","Green", "Yellow", "Blue", "Orange"};
//show array using for each loop.
for(String col : colors)
{
System.out.println(col);
}
}
}
//In the above example, values of colors will assign to the variable col one by one.